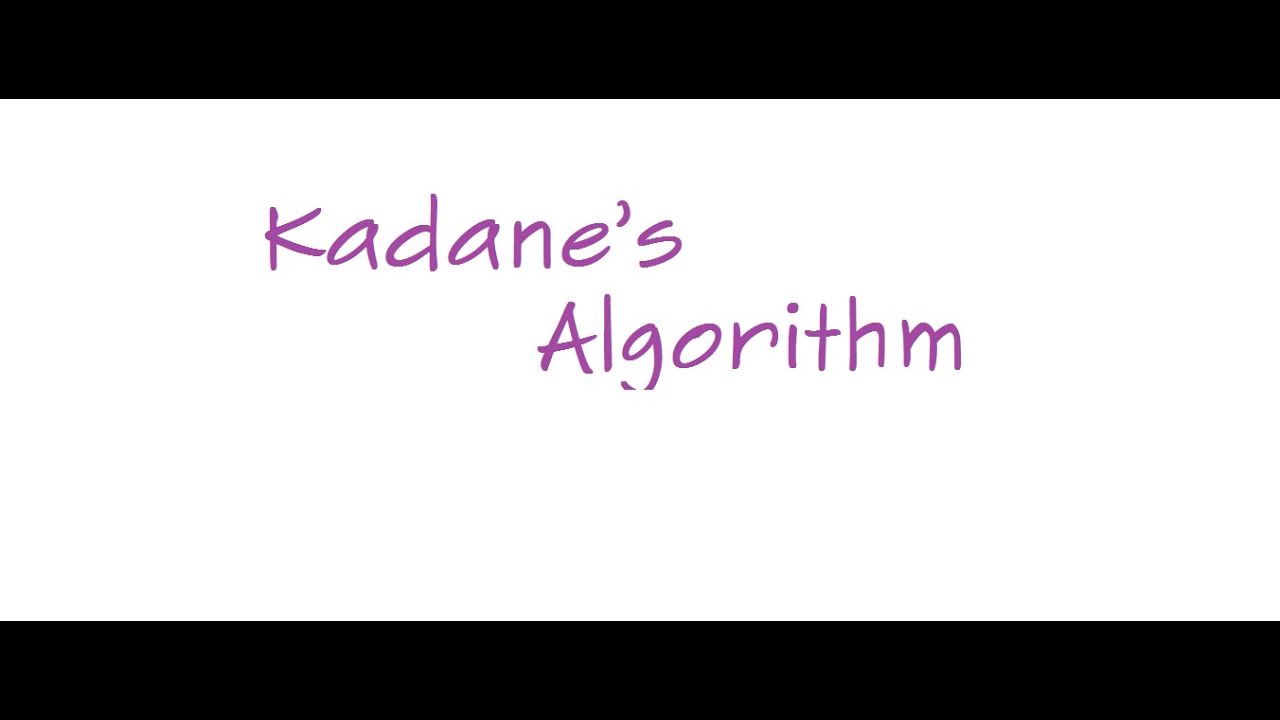
Asked in :
Metlife, Housing.com ,Hike, Flipkart, FactSet, D-E Shaw ,Amazon,Accolite, Snapdeal, Samsung ,PayU , Paytm ,Oracle, Ola,Microsoft, Morgan Stanley
Question:Given an array containing both negative and positive integers. Find the contiguous sub-array with maximum sum.
Input:
The first line of input contains an integer T denoting the number of test cases. The description of T test cases follows. The first line of each test case contains a single integer N denoting the size of array. The second line contains N space-separated integers A1, A2, ..., AN denoting the elements of the array.
Output:
Print the maximum sum of the contiguous sub-array in a separate line for each test case.
Constraints:
1 ≤ T ≤ 200
1 ≤ N ≤ 100
-100 ≤ A[i] <= 100
Example:
Input
2
3
1 2 3
4
-1 -2 -3 -4
Output
6
-1
Code:
import java.io.*;
// Java program to print largest contiguous array sum
import java.util.*;
class Solution
{
public static void main (String[] args)
{
Scanner sc = new Scanner(System.in);
int m = sc.nextInt();
for(int i=0;i<m;i++){
int n = sc.nextInt();
int [] arr = new int[n];
for(int j=0;j<n;j++){
arr[j]= sc.nextInt();
}
System.out.println(maxSubArraySum(arr));
}
}
static int maxSubArraySum(int a[])
{
int size = a.length;
int max_so_far = Integer.MIN_VALUE, max_ending_here = 0;
for (int i = 0; i < size; i++)
{
max_ending_here = max_ending_here + a[i];
if (max_so_far < max_ending_here)
max_so_far = max_ending_here;
if (max_ending_here < 0)
max_ending_here = 0;
}
return max_so_far;
}
}
Comments
Post a Comment