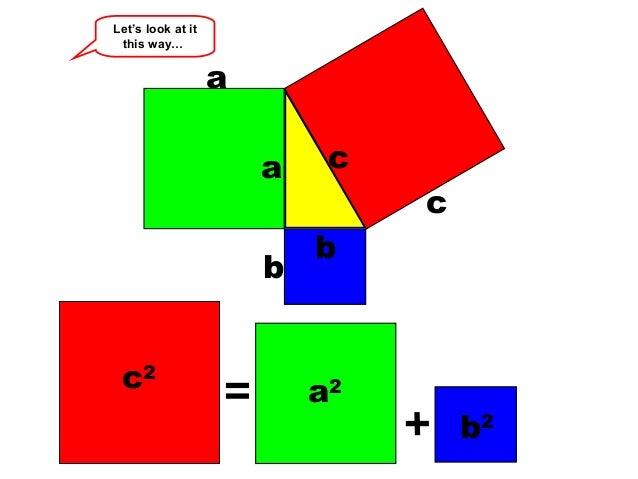
Question:
Given an array of integers, write a function that returns true if there is a triplet (a, b, c) that satisfies a2 + b2 = c2.
Input:
The first line contains 'T' denoting the number of testcases. Then follows description of testcases.
Each case begins with a single positive integer N denoting the size of array.
The second line contains the N space separated positive integers denoting the elements of array A.
Output:
For each testcase, print "Yes" or "No" whtether it is Pythagorean Triplet or not.
Constraints:
1<=T<=50
1<=N<=100
1<=A[i]<=100
Example:
Input:
1
5
3 2 4 6 5
Output:
Yes
The first line contains 'T' denoting the number of testcases. Then follows description of testcases.
Each case begins with a single positive integer N denoting the size of array.
The second line contains the N space separated positive integers denoting the elements of array A.
Output:
For each testcase, print "Yes" or "No" whtether it is Pythagorean Triplet or not.
Constraints:
1<=T<=50
1<=N<=100
1<=A[i]<=100
Example:
Input:
1
5
3 2 4 6 5
Output:
Yes
Solution:
import java.util.*;
import java.lang.*;
import java.io.*;
import java.math.*;
class GFG {
public static void main (String[] args) {
//code
Scanner sc = new Scanner (System.in);
int m = sc.nextInt();//no of test cases
for (int i=0;i<m;i++){
int n = sc.nextInt();//size of array
int []arr= new int [n];
for (int j=0;j<n;j++){
arr[j]= sc.nextInt();
}
check(arr);
}
}
public static int check(int [] arr){
Arrays.sort(arr);
double [] ar = new double[arr.length];
for (int i=0;i<arr.length;i++){
ar[i]=Math.pow(arr[i],2);
}
int count =0;
for (int j=0;j<ar.length-2;j++){
if(ar[j]+ar[j+1]==ar[j+2]){
count+=1;;
}
}
if (count>0){
System.out.println("True");
}
else System.out.println("false");
return -1;
}
}
Enter your Test Inputs:
2
5
3 2 4 6 5
3
3 4 7
Output:
True false
Comments
Post a Comment